Test-Driven Development
According to Wikipedia, "Test-driven development (TDD) is a software development process relying on software requirements being converted to test cases before software is fully developed, and tracking all software development by repeatedly testing the software against all test cases. This is opposed to software being developed first and test cases created later."
Test-driven development goes hand in hand with agile methodology. Here we follow the TDD flow to implement operations of Sql2oCourseDao
which involves writing unit tests for each operation before its implementation.
Unit Testing and JUnit
A "unit test" is a term used in software engineering to refer to a test written to examine a unit — the smallest piece of code that can be logically isolated in a system. In most programming languages, that unit is a function or a method.
We will use a Java library called JUnit to write and run tests. JUnit provides annotations to write tests using the familiar structure and syntax of Java classes and methods but treat them as "tests" once annotated as one.
The library is included by default in an IntelliJ Gradle Java project. The dependencies are added to gradle.build
:
testImplementation 'org.junit.jupiter:junit-jupiter-api:5.6.0'
testRuntimeOnly 'org.junit.jupiter:junit-jupiter-engine'
By convention, the tests are separated from the main files and kept in the src/test/java
folder. Add the following class to this folder, in your project.
import org.junit.jupiter.api.Test;
class Sql2oCourseDaoTest {
@Test
void doNothing() {
}
}
IntelliJ comes equipped with a plugin that facilitates running JUnit tests. You can click on the green play arrow next to each test to run it.
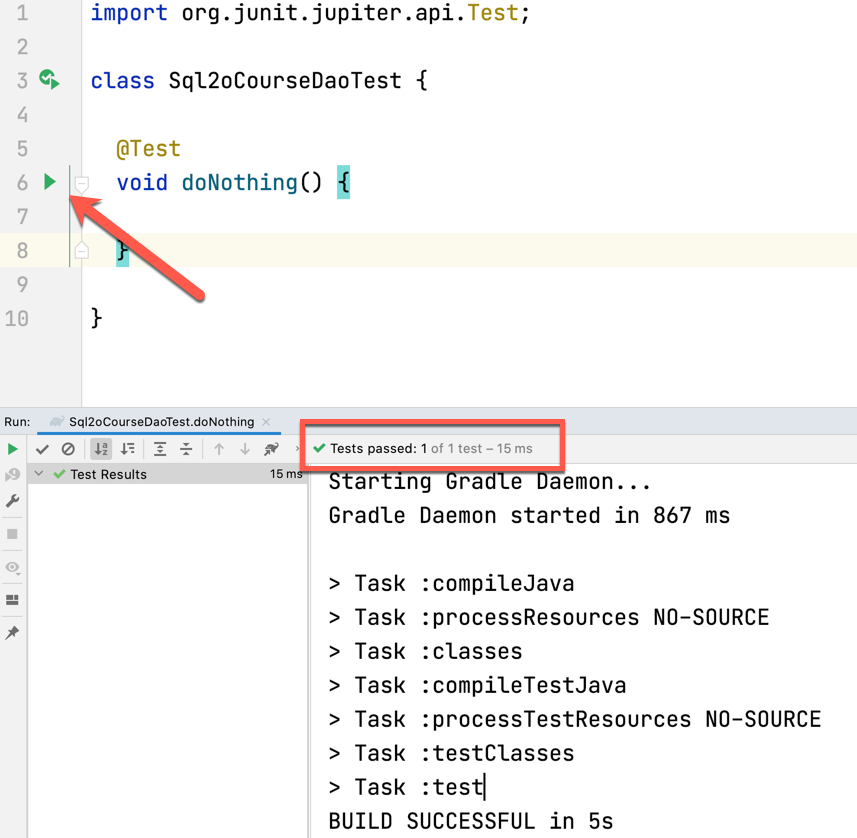
Unit Testing is just one of the many different software testing strategies. You can read more on Software Testing at Software Testing Fundamentals Website.