Connect to the SIS API
We have all the bits and pieces of our application in place, except for actually searching for courses! Let's connect to the SIS API and retrieve the courses matching a given query.
We will use Unirest for communicating with the SIS API from within our Java application. Add the following to the dependencies clause of build.gradle
:
implementation 'com.konghq:unirest-java:3.11.09'
Open the Gradle tool window and refresh the Gradle project.
Open WebServer.java
and add the following lines to the top of the body of the main
method:
final String KEY = System.getenv("SIS_API_KEY");
Unirest.config().defaultBaseUrl("https://sis.jhu.edu/api");
Make sure to add your SIS API KEY as an environment variable in IntelliJ run configuration.
Delete the sample courses we have had created earlier:
- List<Course> courses = new ArrayList<>();
- courses.add(new Course("EN.500.112","GATEWAY COMPUTING: JAVA"));
- courses.add(new Course("EN.601.220","INTERMEDIATE PROGRAMMING"));
- courses.add(new Course("EN.601.226","DATA STRUCTURES"));
- courses.add(new Course("EN.601.229","COMPUTER SYSTEM FUNDAMENTALS"));
- courses.add(new Course("EN.601.231","AUTOMATA and COMPUTATION THEORY"));
Finally, update the GET rout method for /search
as follows:
get("/search", (req, res) -> {
String query = req.queryParams("query");
+ Set<Course> courses = Unirest.get("/classes")
+ .queryString("Key", KEY)
+ .queryString("CourseTitle", query)
+ .asObject(new GenericType<Set<Course>>() {})
+ .getBody();
Map<String, Object> model = Map.of("query", query, "courses", courses);
return new ModelAndView(model, "search.hbs");
}, new HandlebarsTemplateEngine());
I'm storing courses in a Set
because the SIS API data contains many duplicates (each section of each course from Spring 2009 until now will appear as a separate "class").
Run the application and give it a try!
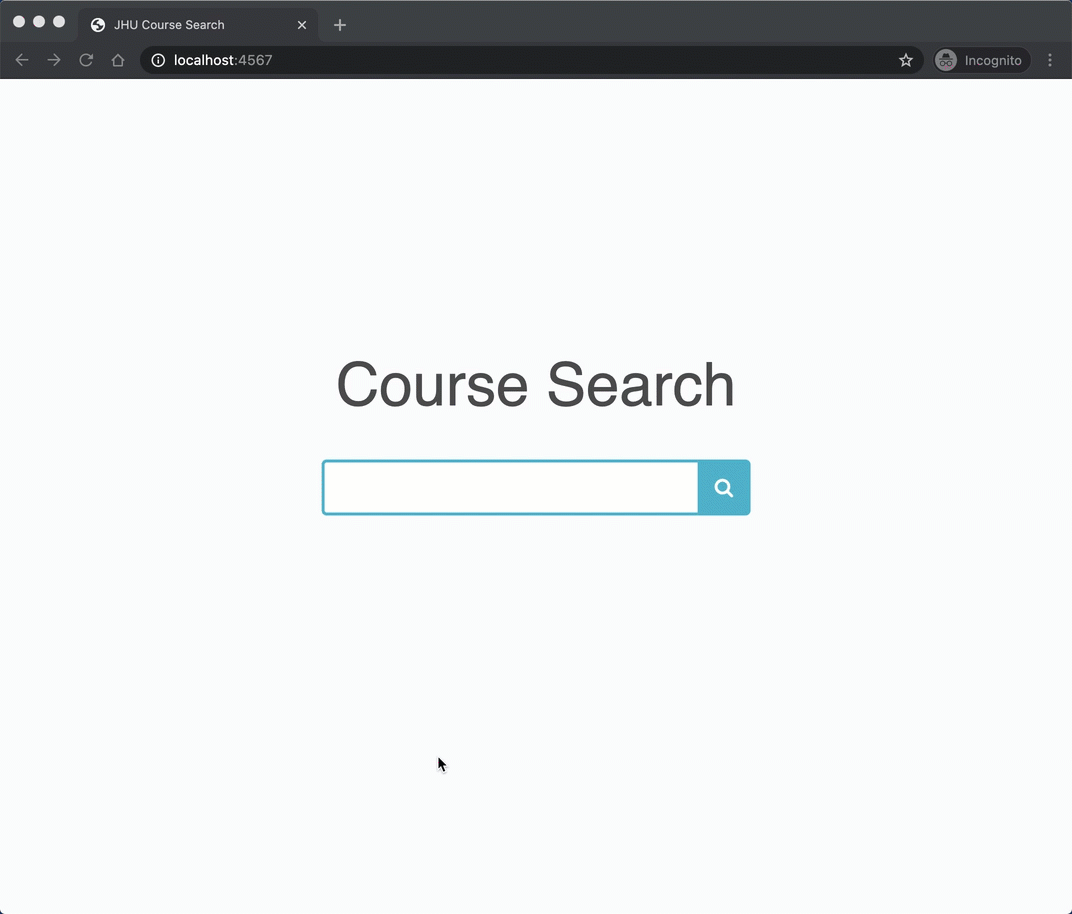