Search
Here is how our app behaves when a user searches for a course:
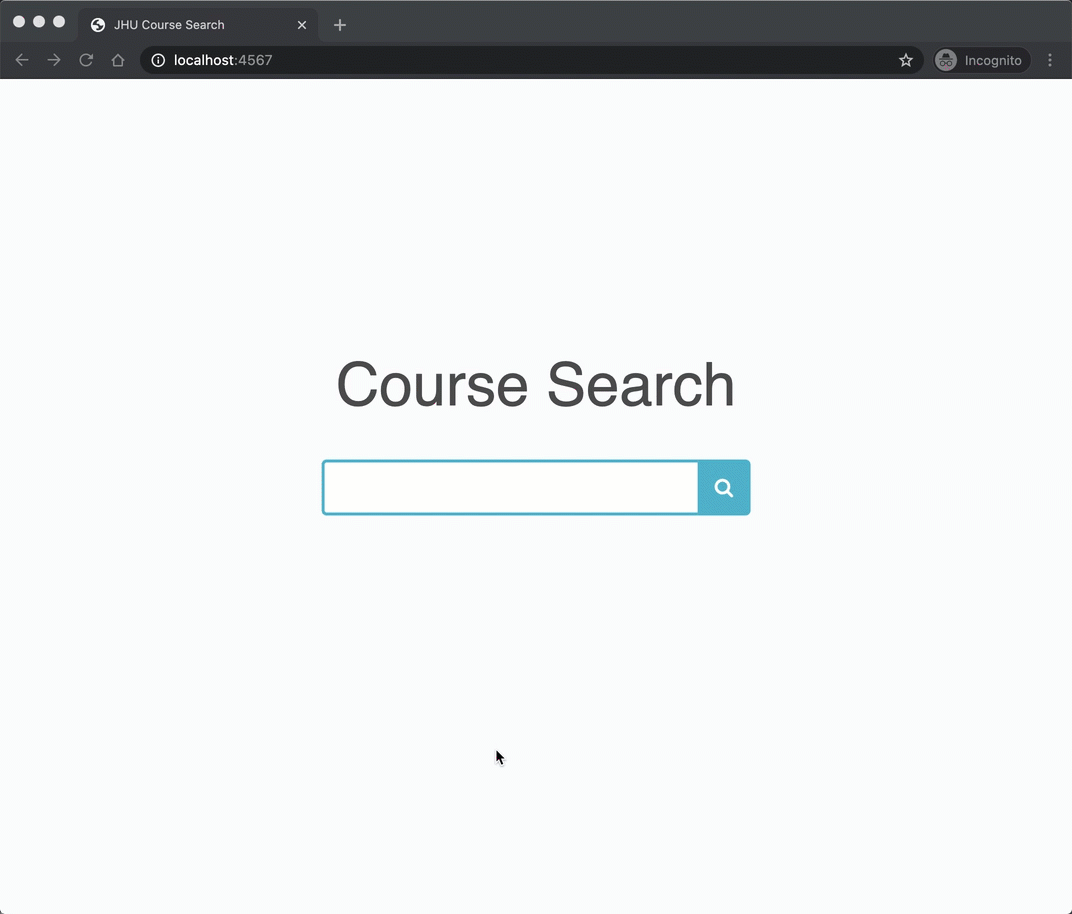
Let's trace this in the code; looking at index.hbs
, notice the "action" of the form:
<form class="search" action="/search" method="post">
<input type="text" class="searchTerm" placeholder="" name="query">
<button type="submit" class="searchButton">
<i class="fa fa-search"></i>
</button>
</form>
It sends a HTTP POST request to the endpoint /search
. Moreover, it provides a payload (body) containing:
{
"query": "whatever user typed in the input textbox"
}
To manage this, we need to add a route method in WebServer.main
:
post("/search", (req, res) -> {
String query = req.queryParams("query");
res.redirect("/search?query=" + query);
return null;
}, new HandlebarsTemplateEngine());
Notice in the route method above, the route handler simply collects the search query and it redirects the request to route /search
providing the search term as a query parameter.
We can update the route method for /search
GET request to capture the query parameter and pass it to handlebars engine.
get("/search", (req, res) -> {
+ String query = req.queryParams("query");
+ Map<String, Object> model = Map.of("query", query, "courses", courses);
- Map<String, Object> model = Map.of("courses", courses);
return new ModelAndView(model, "search.hbs");
}, new HandlebarsTemplateEngine());
Finally, update the search.hbs
to show the search query in the search input:
- <input type="text" class="searchTerm" placeholder="" name="query">
+ <input type="text" class="searchTerm" placeholder="" name="query" value="{{query}}">
Here is the result:
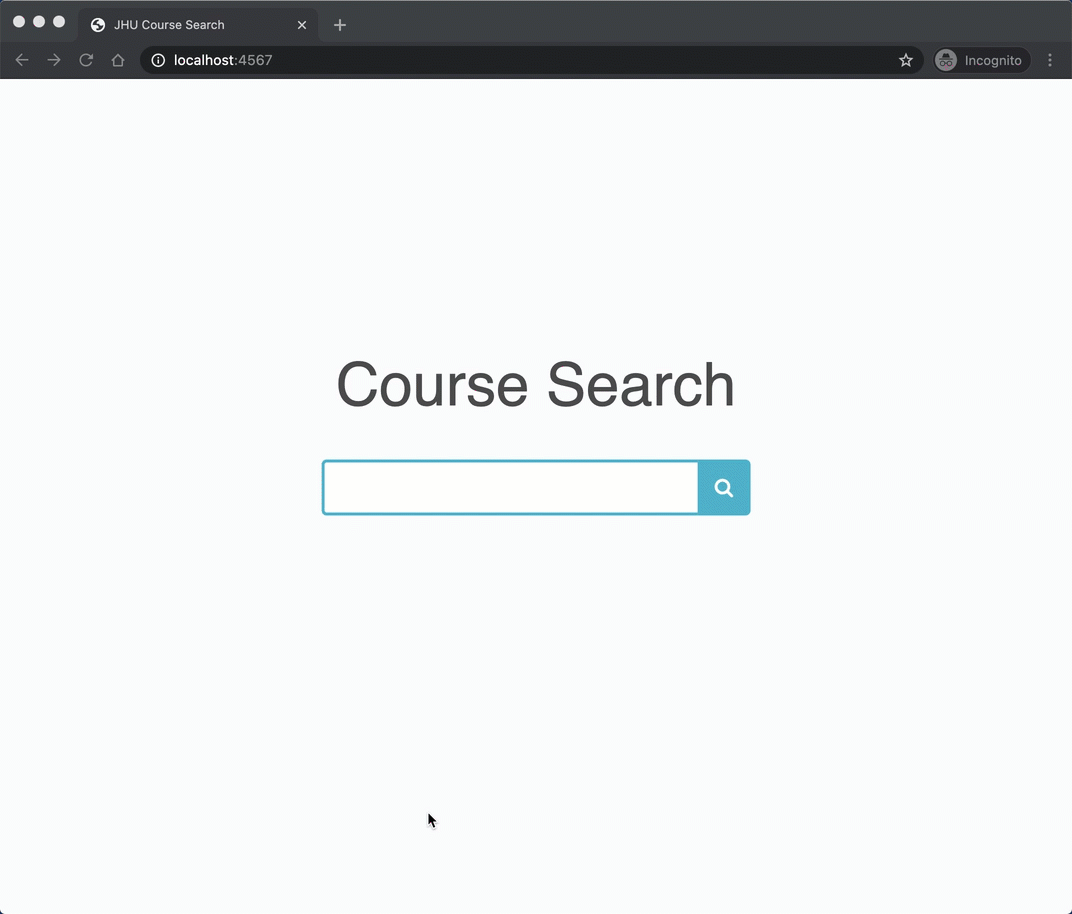